If you are reading this and if you are not me, this is not a tutorial or guideline, rather a scratchpad for myself to use as a reference for my future projects.
Basics
- GNU Toolchain
- GNU Autotools : Easy way to manage and package source code. “./configure >> make >> make install >> make uninstall”. There are three primary components : automake, autoconf, make
- GNU Binutils
- GNU Bison
- GNU C Library
- GNU Compiler Collection
- GNU Debugger
- GNU m4
- GNU make
2. Das U-Boot
https://github.com/u-boot/u-boot
3. QEMU
QEMU is an open source emulator. It can emulate a target without needing any hardware virtualization. It can provide userspace API virtualization for Linux kernel, so binaries compiled agains one ABI, can run on a host with a different ABI. (RiscV on Linux x86_64 API for instance).
There is no hardware emulation. It is just CPU and syscall emulation.
The Target
The first target is GW5AST-LV138FPG676AC2/I1. Besides 138K LUT4s (yes it is huge!), 2.5Gbps MIPI DPHY, PCIe 2.0 and 12.5Gbps transceivers, there is an Andes AE350_SOC A25 series RiscV core. It is not a soft IP core, it is a hard-core processor. APB and AHB slave buses extend to the FPGA logic. AHB master bus extends to the FPGA logic.
The SoC consists of the following blocks:
- Core system : RiscV Core, PLIC, PLMT, debug, clock gating, reset, I-Cache, D-Cache, ILM, DLM
- Memory system : Instruction and data memory
- Bus Peripheral system : AHB bus and APB bus peripherals.
- AHB Bus Peripherals
- 64-bit Extended AHB Master
- 32-bit Extended AHB Slave
- AHB2APB Bridge
- APB bus peripherals
- I2C, PIT, SPI, UART1, UART2, GPIO, WDT, RTC, SMU, DMAC, 32-bit extended APB slave
- AHB Bus Peripherals
Features
- 5-stage in-order execution pipeline
- Max 800MHz clock
- Machine, Supervisor, User modes
- Hardware multiplier and divider
- Hardware performance monitors
- Hardware stack protection extension
- Memory management
- Physical memory protection entries
- 32KB I-Cache and D-Cache, LRU algorithm supported
- 64KB ILM and DLM embedded
- DMA
Development Environment and Tools
The vendor provides an SDK called “RDS Software”; it is based on Andes IDK (integrated development kit), but it targets bare metal, FreeRTOS, and Zephyr applications. The libraries are “newlib” and “mculib” in this context. The vendor SDK (RDS) lists the following as a toolchain while creating a new project:
- nds32le-elf-mculib-v5 : MCUlib is smaller C library (smaller than newlib), for MCU applications
- nds32le-elf-mculib-v5d : MCUlib is smaller C library (smaller than newlib), for MCU applications
- nds32le-elf-mculib-v5f : MCUlib is smaller C library (smaller than newlib), for MCU applications
- nds32le-elf-newlib-v5 : C library for embedded systems
- nds32le-elf-newlib-v5d C library for embedded systems
- nds32le-elf-newlib-v5f : C library for embedded systems
- nds32le-linux-glibc-v5d : Standard C library for Linux
Currently, the RDS (RiscV_AE350_SOC_RDS_V1.3_win) is only available for Windows. The EDA tool has both Linux x86 and Windows versions. This is about the software development tools (RDS). The Windows installer installs OpenOCD binaries too. It has an Eclipse-based IDE and in the background there is Cygwin. As of the toolchain, these are the only archive packages in the installer ZIP:
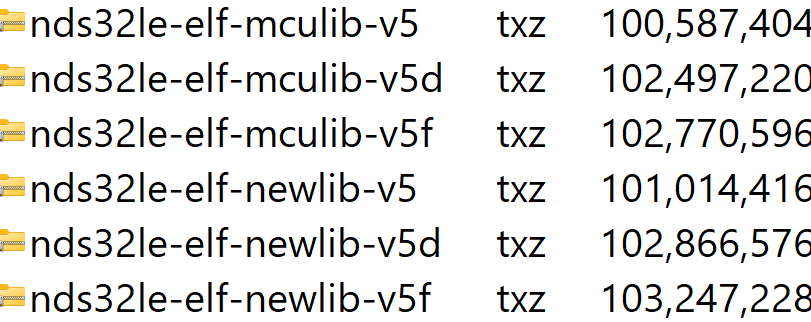
nds32le-linux-glibc-v5d is probably not included in the installer. We will come back to this later.
In the Demo Projects folder there are dedicated example folders.

I have never heard of rtthread before. Here is some intro from the readme: RT-Thread is an open source IoT operating system from China, which has strong scalability: from a tiny kernel running on a tiny core, for example ARM Cortex-M0, or Cortex-M3/4/7, to a rich feature system running on MIPS32, ARM Cortex-A8, ARM Cortex-A9 DualCore etc.
And there are zephyr examples but it is not relevant for now.
nds32le-linux-glibc-v5d
This toolchain and other nds32le, nds64le toolchains with corresponding ABIs are available in the SoC core vendor (Andes) GitHub repository, nds-gnu-toolchain:
https://github.com/andestech/nds-gnu-toolchain
Toolchain | ARCH | ABI | CPU | TARGET |
---|---|---|---|---|
nds32le-linux-glibc-v5 | rv32imacxandes | ilp32 | andes-25-series | riscv32-linux |
nds32le-linux-glibc-v5d | rv32imafdcxandes | ilp32d | andes-25-series | riscv32-linux |
nds64le-linux-glibc-v5 | rv64imacxandes | lp64 | andes-25-series | riscv64-linux |
nds64le-linux-glibc-v5d | rv64imafdcxandes | lp64d | andes-25-series | riscv64-linux |
Let’s break down the architecture names;
rv32imacxandes (and followed by the other extension marks not included in this particular core)
Mark | Description |
---|---|
rv32 | RISV-V (RV), 32-bit architecture |
i | Base Integer instruction set (required for all RISC-V processors) |
m | Integer Multiplication & Division extension |
a | Atomic instructions extension (e.g., for multithreading/locks) |
c | Compressed instructions (16-bit compressed instruction support for reduced code size) |
xandes | Custom Andes extensions, non-standard |
f | Single-precision Floating Point (IEEE 754) |
d | Double-precision Floating Point |
q | Quad-precision Floating Point |
b | Bit Manipulation (efficient bitwise operations) |
v | Vector processing (for SIMD operations, AI, HPC) |
h | Hypervisor support (for virtualization) |
s | Supervisor Mode (needed for running Linux) |
u | User Mode (used for applications) |
What is ABI?
Short for Application Binary Interface. The ABI defines how the arguments are passed to a function, how a return value is returned (via which CPU register for instance), use of registers, stack frame layout, how the functions are placed in a library binary and how to locate and execute a function from a library.
Register | ABI Name | Description | Saver |
---|---|---|---|
x0 | zero | Hard-wired to zero | – |
x1 | ra | Return address | Caller >>> |
x2 | sp | Stack pointer | Callee <<< |
x3 | gp | Global pointer | — |
x4 | tp | Thread pointer | — |
x5-7 | t0-2 | Temporaries | Caller >>> |
x8 | s0/fp | Saved register/frame pointer | Callee <<< |
x9 | s1 | Saved register | Callee <<< |
x10-11 | a0-1 | Function arguments/return values | Caller >>> |
x12-17 | a2-7 | Function arguments | Caller >>> |
x18-27 | s2-11 | Saved registers | Callee <<< |
x28-31 | t3-6 | Temporaries | Caller >>> |
For ABI, there are to kinds of registers: calle-save and caller-save. The callee-saved registers need to be saved and restored by the function being called (callee). The registers defined as caller-save are allowed to be changed freely by the function being called.
sudo apt-get install opensbi
find / -name “opensbi*fw_dynamic.bin”
qemu-system-riscv32 -machine virt -nographic -bios /usr/share/qemu/opensbi-riscv64-generic-fw_dynamic.bin -kernel test
Since the goal is building uboot, we need a Linux toolchain. Here is some experimentation with qemu and the toolchain.
This is how the toolchain is build for Linux
./build_linux_toolchain.sh
There is also the build script “build_elf_toolchain.sh”; for bare-metal. It is not in the scope yet.
This is how the build_linux_toolchain.sh look like:
TARGET=riscv32-linux
PREFIX=pwd/nds32le-linux-glibc-v5d
ARCH=rv32imafdcxandes
ABI=ilp32d
CPU=andes-25-series
XLEN=32
BUILD=pwd/build-nds32le-linux-glibc-v5d
SYSROOT=${PREFIX}/sysroot
TARGET_CC=${PREFIX}/bin/${TARGET}-gcc
TARGET_CXX=${PREFIX}/bin/${TARGET}-g++